Perfect Number | single number | 1 to 100 | in c# with source code | output with explanation
In this program i have shown how to check whether entered number is perfect number or not.
Logic i have developed is so simple .Anyone can learn easily.
so first i will show you how to check...
Perfect Number | program for single number | 1 to 100 | in c# with source code | output
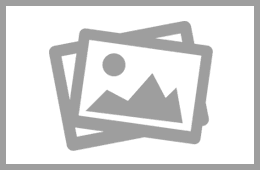