/* use of token pasting operator with Output *///Token pasting operator is used to concatenate two tokens into a single token.#include#define PASTE(a,b) a##b#define MARKS(sub) marks_##subint main(){int k1=15,k2=60;int marks_os= 95, marks_ds=99;//converting statement below to printf("%d %d",k1,k2)printf("%d...
Token pasting operator in c language
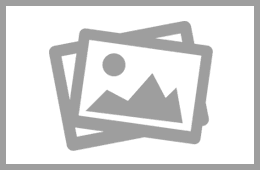