C Language is First Programming Language.
I Can say it is base of all Programming Language.
To develop the code in Java ,c# , VB , c++ ,Or any Language we need C.
I am not saying that only C is language where you can apply your all logic but if you will apply then it
will...
How to develop logic in c programming language with programs
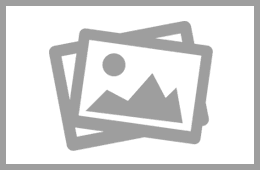