cmd.Parameters.Add("@stud_rollno", txtstudroll); cmd.Parameters.Add("@paidfee", txtpaid); cmd.Parameters.Add("@remain_fee",...
No mapping exists from object type System.Web.UI.HtmlControls.HtmlInputText to a known managed provider native type.
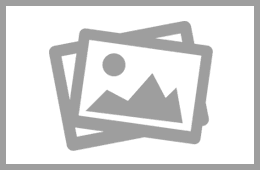